7.3 - Loading and spawning asset bundles in game
How to make the mod locate the asset bundle file?
First create our mod project as usual, then we create a new class with whatever name we want, like TankPrefab
.
// our new TankPrefab class created inside the TankPrefab.cs file
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace TankModExample;
public class TankPrefab
{
}
Then we need to add using SonsSdk.Attributes;
at the top and do as below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using SonsSdk.Attributes;
using UnityEngine;
[AssetBundle("tank")] // tank is the name we gave to the asset bundle in unity bottom right corner
public class TankPrefab
{
[AssetReference("Leopard2")] // Leopard2 is the name of the prefab in Unity
public static GameObject Tank { get; set; }
}
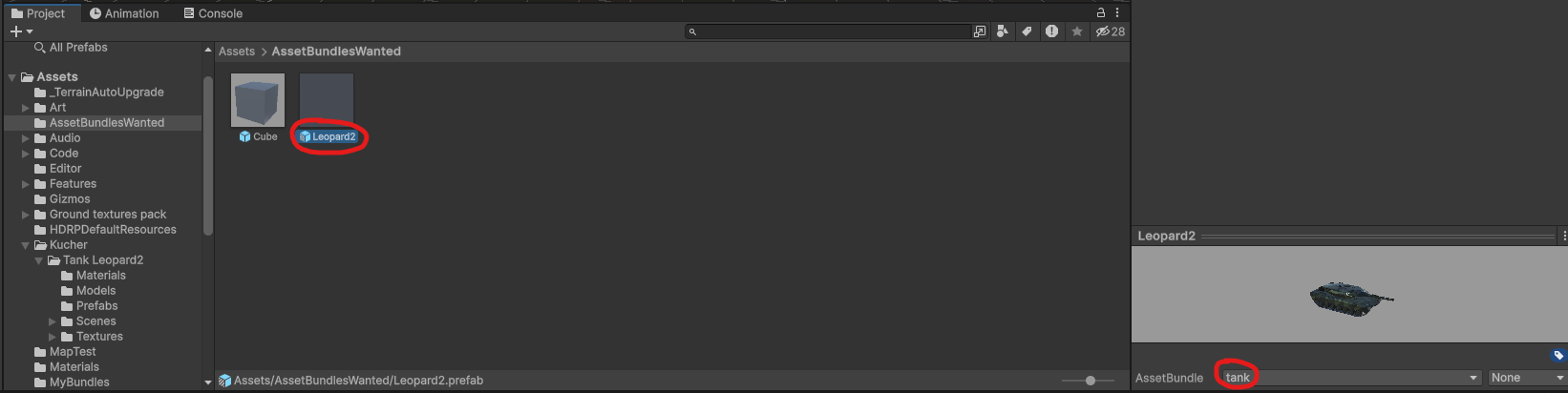
We now need to get the asset bundle file we made in previous lesson (the one which has no extension) and place it inside the mod folder of our mod like so:
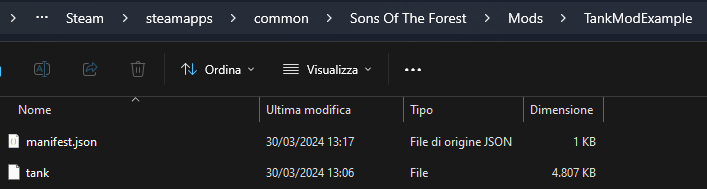
Spawning the asset bundle in game
We can now spawn the asset bundle in game using the code below wherever we want:
// spawning the assetbundle at the given positon and rotation
var tank = UnityEngine.Object.Instantiate(TankPrefab.Tank, SonsTools.GetPositionInFrontOfPlayer(4, 2), Quaternion.identity);
// tank is our spawned GameObject
If upon spawning the asset bundle you see it's completely black, follow the next lesson.